datetime:2019/5/21 16:23
author:nzb
创建刻度盘
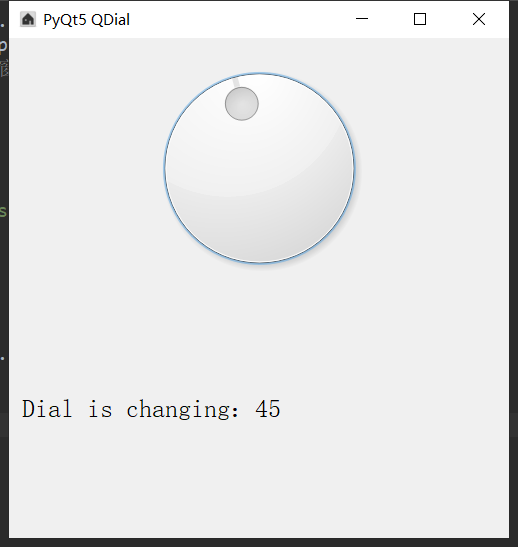
import sys
from PyQt5.QtWidgets import QWidget, QApplication, QLabel, QGroupBox, QCheckBox, \
QHBoxLayout, QFrame, QScrollArea, QFormLayout, QPushButton, QVBoxLayout
from PyQt5.QtWidgets import QDial
from PyQt5 import QtGui, QtCore
from PyQt5.QtCore import Qt
class UI_demo(QWidget):
"""用户界面"""
def __init__(self):
super().__init__()
self.title = 'PyQt5 QDial'
self.left = 600
self.top = 200
self.width = 500
self.height = 500
self.iconName = '../img/home.ico'
self.initWindow()
def initWindow(self):
self.setWindowIcon(QtGui.QIcon(self.iconName))
self.setGeometry(self.left, self.top, self.width, self.height)
self.setWindowTitle(self.title)
vbox = QVBoxLayout()
self.label = QLabel(self)
self.label.setFont(QtGui.QFont('Sanserif', 15))
self.dial = QDial()
self.dial.setMinimum(0)
self.dial.setMaximum(100)
self.dial.setValue(30)
self.dial.valueChanged.connect(self.dial_changed)
vbox.addWidget(self.dial)
vbox.addWidget(self.label)
self.setLayout(vbox)
self.show()
def dial_changed(self):
getValue = self.dial.value()
self.label.setText("Dial is changing:" + str(getValue))
if __name__ == "__main__":
app = QApplication(sys.argv)
ex = UI_demo()
sys.exit(app.exec_())